Posted by Nick Sieger
Sat, 06 Oct 2007 12:04:00 GMT
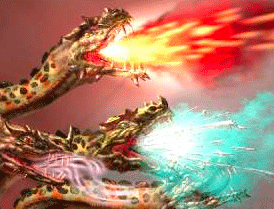
On September 19, Craig and I presented our talk at RailsConf, which appears to have been well received. It went off mostly without a hitch, if it wasn’t for a couple of hiccups in the demos. I apparently didn’t practice them enough, because a couple of critical steps were either missed or I did them out of order and confused myself. But that’s ok, because I’m releasing the demo steps, source and slides here so you can try them out for yourself.
So, download the zip and follow along. The contents look like this:
1-active-resource-basics.txt
2-make-resourceful.txt
3-atom-roller.txt
4-service-chatter.txt
RailsConfEurope-Hydra.pdf
demo/
demo-baked/
The demo steps are in the text files; I’d recommend going in the order specified. It turns out the third isn’t really a demo but more of a code review, because it also requires you to have Roller set up and I’m not going into those details for now. If you want to just run the demos, skip to demo-baked
, the finished product.
For those of you who didn’t see the talk, here’s the basic message.
Look at your basic MVC Rails app.
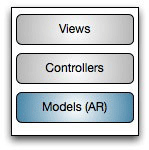
Why not consider spliting it into two? ActiveResource allows you to access a RESTful resource in your Rails application like it was just another model.
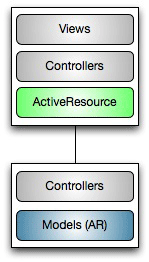
This might seem like overkill for a simple application, but what if you had an e-commerce application domain like this?
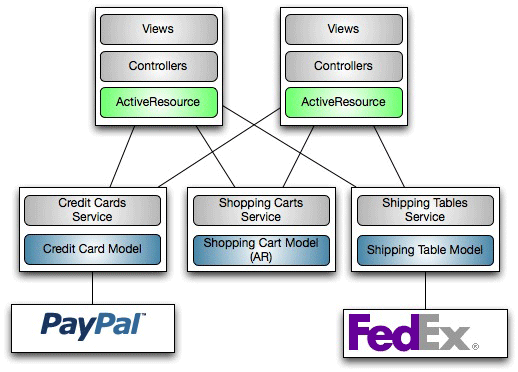
Splitting up your code into separate Rails applications encourages encapsulation, reduces potential coupling, and gives you more flexible deployment options. Basing interactions upon REST and HTTP means that you can more easily mash up data or create caching strategies, given proper usage of ETags/Last-Modified and/or cache-control headers. The great thing is that existing HTTP reverse proxies can be used without having to mix the caching code in with your application code.
In the application we’re building, we have a number components that are not Rails-based. To expose them to our environment, we’ve taken the strategy of exposing a simple REST web service for the component, and then it can be consumed by the other applications using ActiveResource. The REST web service can either be implemented in the component’s native language/technology, or in some cases we’ve written a wrapper service in Rails since Rails makes it so easy to build REST interfaces. In that case, Rails is pure integration -- RESTful glue.
The idiom that makes this all possible is the uniform interface. In HTTP, this means addressability (each resource gets a unique URI) coupled with the HTTP method verbs HEAD, GET, POST, PUT, and DELETE. Inside your Rails applications, it’s the ActiveRecord interface. If you keep your controllers skinny, you can boil the interface down to the following set of methods (in this case, for the prototypical blog post model):
Post.new/Post.create
Post.find
@post.save
@post.update_attributes
@post.errors
@post.destroy
And in fact, this is precisely what ActiveResource provides, and it’s enabled by duck-typing. Walking through the demos illustrates this pretty well, as you’ll basically swap ActiveRecord for ActiveResource with no noticeable difference, all the way down to validation errors in the scaffolded forms (which I was unable to demo in the talk due to the hiccups).
We’ve found that when you’re making RESTful web services, the controllers largely become boilerplate because of the uniform interface. make_resourceful
has been a boon in that regard, as the demos also show. There are several plugins that help you DRY up your controllers (other approaches include resources_controller
), so you have some choices there.
We mentioned some drawbacks in our experience with ActiveResource, which have largely been addressed for the upcoming Rails 2.0 release.
Finally, we noted that deployment could be a pain with so many Rails applications to keep running. To that end, we are leveraging JRuby and Glassfish to make this a non-issue, as we simply WAR up our Rails applications with warbler and let Glassfish take care of the rest. Performance is still an open question, but we plan to roll up our sleeves and make sure this combination really hums.
Enjoy the demos! Feel free to drop me an email if you have any questions or troubles with them.
Tags railsconf, railsconfeurope2007 | 5 comments
Posted by Nick Sieger
Tue, 18 Sep 2007 08:14:00 GMT
Rebel With a Cause
Rails is no longer the James Dean character, looking outward, trying to convince you of something. It’s no longer about a rebellion or a revolution. Instead, Rails is settling in as a passionate, inward-looking craftsperson.
After the rebellion, settle in and enjoy the results of your work. David hasn’t been working directly on Rails too much, instead he’s been enjoying it.
And it’s not about David anymore. It’s about You (cue the Time Person of the Year cover). David wants Rails to be more friendly to newcomers.
Report #12: Verified Patches. Encouraging the community to approve patches, rather than limiting the decisions to the core. Opening up Rails. (Also, unfortunately looking a little scarce at the moment. Update: turns out it’s empty because patches are quickly applied, not because there aren’t any submitted!)
David screened the original Rails movie -- showing Apache setup, manually creating databases and tables. He quickly lost patience for it.
Compare to the current state of the art, with everything down to the routes and migrations gets created for you, lowering the barrier to entry (example: a new rake task db:create:all
).
Rails 2.0 is largely about continual improvement and removing the cruft:
- Cookie-based session store as the default
- Routing:
map.root
- Removing the dynamic
scaffold :posts
feature
map.namespace
and script/generate controller admin::posts
*.html.erb
files with the MIME type and renderer baked into the filename, since the two are now mutually exclusive.
- Automatically named partials with
render :partial => @posts
resolving to _post.html.erb
- Namespacing and named routes:
mop.namespace :admin do |admin|
admin.resources :posts
end
link_to 'Show', [:admin, post]
class Admin::PostsController < ApplicationController
before_filter :ensure_administrator
private
def ensure_administrator
authenticate_or_request_with_http_basic("Blog Admin") do |user,pass|
username == "dhh" && password == "123"
end
end
end
- Custom layouts for specific user agents (say, oh, the iPhone). Views can also be rendered, e.g.,
index.iphone.erb
.
class ApplicationController < ActionController::Base
before_filter :adjust_format_for_iphone
def adjust_format_for_iphone
if request.env["HTTP_USER_AGENT"][/iPhone/]
request.format = :iphone
end
end
end
Mime::Type.register "application/x-iphone", :iphone
respond_to do |format|
format.iphone { render :text => "Hello iPhone", :content => Mime::HTML }
end
atom_feed_helper
, a new plugin -- builder for Atom specified in index.atom.builder
<%= yield :head %>
, content_for :head { auto_discovery_link(:atom, formatted_posts_url)}
- Debugger -- allows you to be lazy and leave your
breakpoint
s in your production code, not that you’d actually want to do that. Dumps you into irb where you can inspect variables, etc. but you can also drop down another level to see the call hierarchy, etc.
So when will we see Rails 2.0? The preview release is coming, hopefully before conference end.
Tags railsconf, railsconfeurope2007 | 6 comments
Posted by Nick Sieger
Tue, 18 Sep 2007 08:08:34 GMT
(Written from Dave’s perspective, in the first person, but paraphrased)
What is a relevant topic in Germany? Engineering. Except there’s no such thing as software engineering. The software equivalent of building a bridge is taking a whole lot of dirt that fills in a hole.
So what makes engineering good? I look for elegance and beauty.
Fred Brooks -- Mythical Man-Month. Not a single thing in software engineering has changed in the 30 years since the book was written. Go out and order it tonight if you haven’t read it.
We are privileged, because we get to start with nothing. Anything we can conceive of, we can create. And so, we suffer the same problems as poets suffer.
Writer’s block: a blank page standing in the way of getting started. Painting: a blank canvas without structure or form. Software project: a blank editor buffer.
Leonardo was commissioned to build a statue. But, having never bronzed in his life, he picked up a scrap of paper and drew sketches. He’s prototyping on paper, but in reality he’s prototyping in his mind, getting his brain thinking about what he’s doing.
We could do more of this experimentation. Whiteboard, index cards, we eschew them for scaffolding or other crutches. But we could think of more ideas if we got away from the familiar and tossed around new, fresh ideas.
The idea that we sit down and start coding the application is crazy. We don’t know what it’s supposed to be. Why should we fool ourselves into thinking that this works?
Artists draw cartoons, often a fully rendered version of a painting. If they don’t like the cartoon, it survives, but the final product probably takes a different form.
With Rails we can do rapid end-to-end prototyping. Scaffolding helps with this, and it probably won’t make it into the end result.
So start anyway, and be prepared to throw it away. Write tests, use them as tracer bullets. And act on worry. Listen to the inner voice that tells you when something is wrong.
Sistine Chapel: brilliant example of modularization. It shows you how to conceive of doing a huge project without the fret of focusing on the entire thing.
Another example: comic books. You’re splitting up the product into time slices. Limit how much you do, and leave open the possibility of continuing at a later day. Know when to stop.
Satisfy the customer. Compare portraits to pictures. The best portraits, while not always faithful to the real image of the subject, still achieve the goal of satisfying the customer.
There is art in engineering, and engineering in art. Neither is an either/or proposition. Art and engineering are mutually supportive, in that you can’t have one without another.
With Ruby and Rails, we have a responsibility to uphold. Rails is a canvas, so be an artist. Create something great. But we also should create something beautiful. Sign your name by your work, and take pride in it.
Tags railsconf, railsconfeurope2007 | 3 comments